Mobile Development With Flutter Training
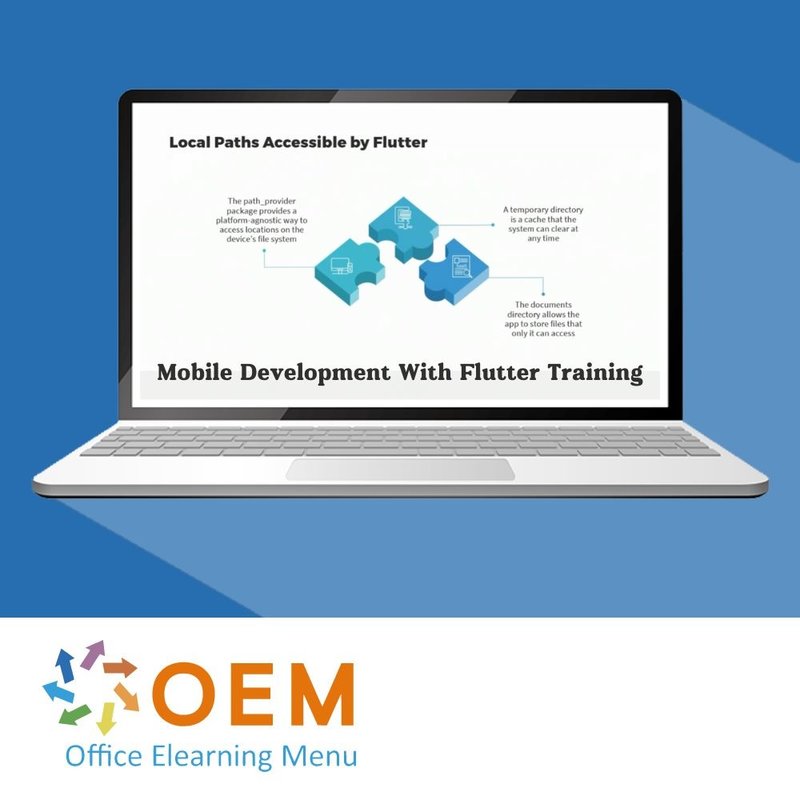
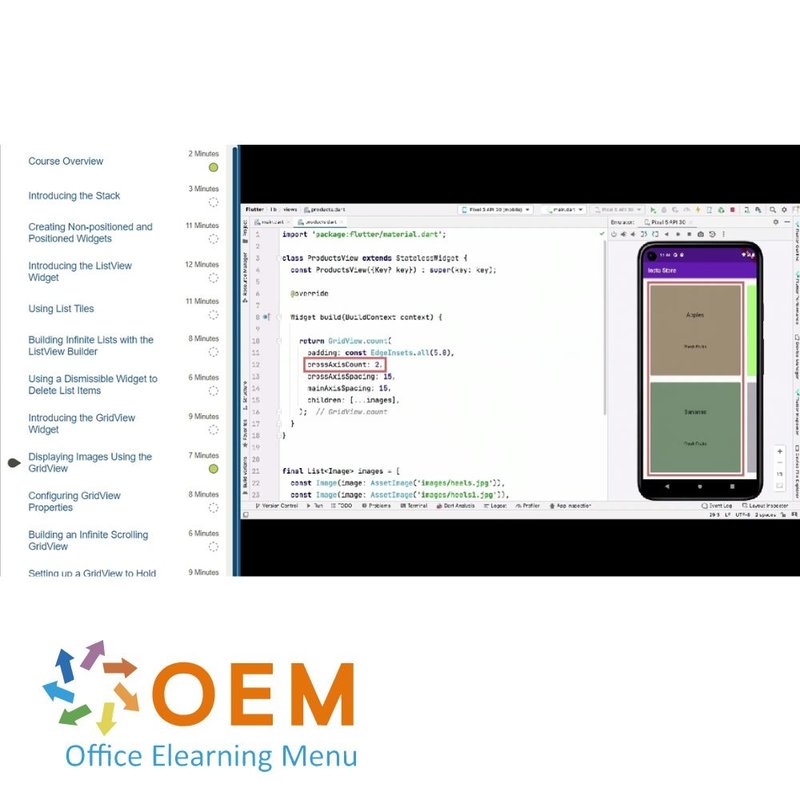
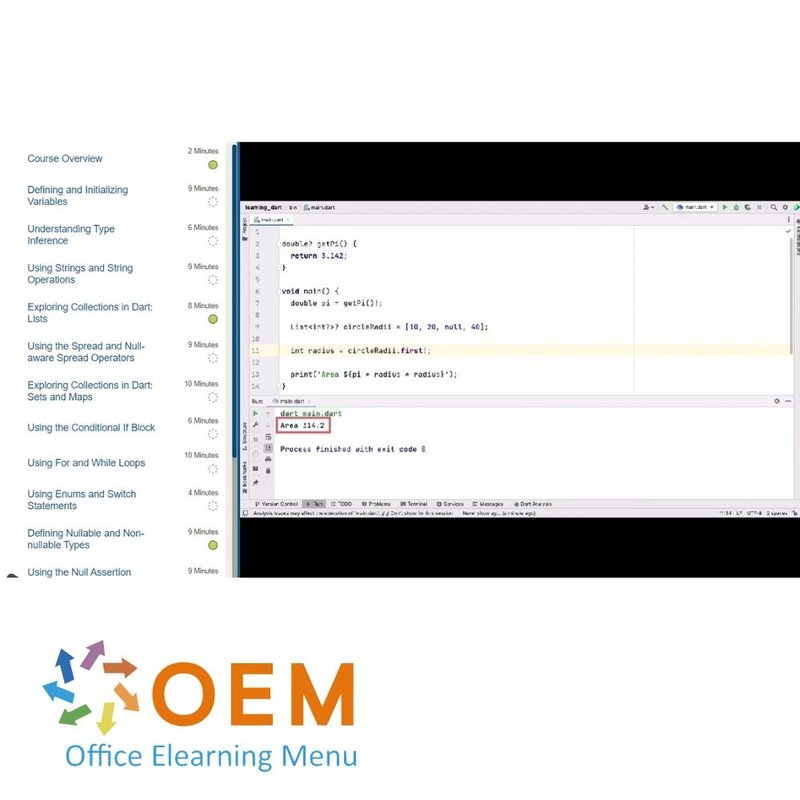
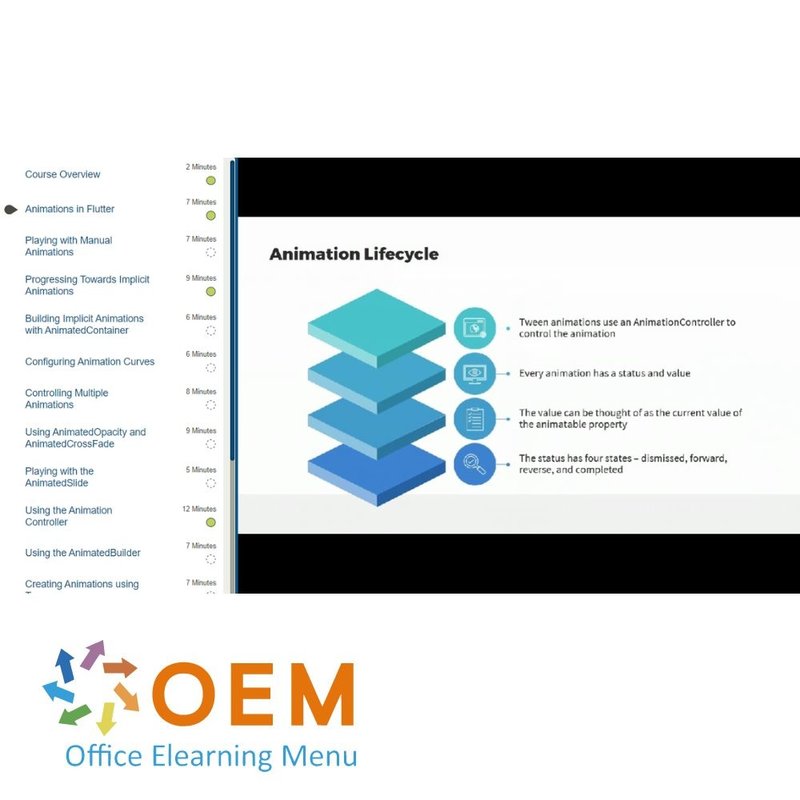
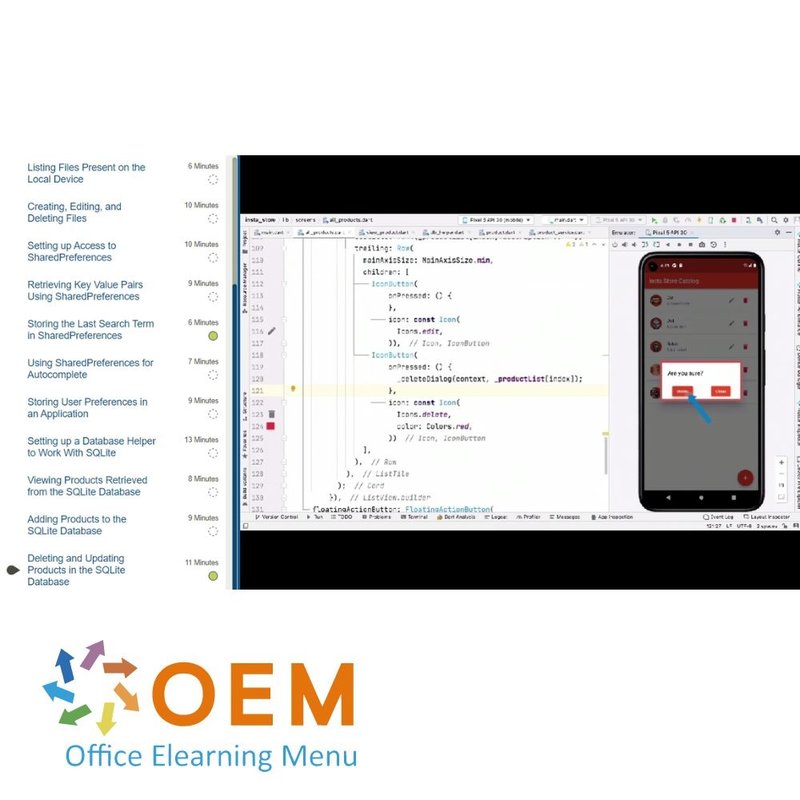
Mobile Development With Flutter Training
Mobile Development With Flutter E-Learning Training Gecertificeerde docenten Quizzen Assessments Tips Tricks Certificate.
Read more- Discounts:
-
- Buy 2 for €194,04 each and save 2%
- Buy 3 for €192,06 each and save 3%
- Buy 4 for €190,08 each and save 4%
- Buy 5 for €188,10 each and save 5%
- Buy 10 for €178,20 each and save 10%
- Buy 25 for €168,30 each and save 15%
- Buy 50 for €158,40 each and save 20%
- Availability:
- In stock
- Delivery time:
- Ordered before 5 p.m.! Start today.
- Award Winning E-learning
- Lowest price guarantee
- Personalized service by our expert team
- Pay safely online or by invoice
- Order and start within 24 hours
Mobile Development With Flutter E-Learning
In recent years application development has become more complex and time-consuming as users expect the same application and functionality to be available across different platforms. The result has been increased adoption of technologies such as Flutter that support cross-platform development allowing organizations to use a single codebase to develop applications across multiple environments. In this course, you will learn how Flutter helps develop cross-platform applications.
This Learning Kit with more than 23 hours of learning is divided into three tracks:
Track 1: Fundamentals of Dart Programming and Flutter Framework
Courses (13 hour +):
Flutter Development: Introducing Flutter & the Dart Programming Language
Course: 1 Hour, 45 Minutes
- Course Overview
- What is Flutter?
- Why Use Flutter?
- Architectural Layers in Flutter
- Flutter Layers for Mobile and Web Platforms
- Flutter vs. Other Frameworks
- The Dart Programming Language
- Introducing DartPad
- Running Simple Programs in DartPad
- Installing the Dart SDK on Mac OS
- Installing the Dart SDK on Windows
- Creating and Running a Command-line App
- JIT and AOT on the Command Line
- Setting up a Console App on IntelliJ
- Course Summary
Flutter Development: Using Variables, Collections, & Functions in Dart
Course: 2 Hours, 10 Minutes
- Course Overview
- Defining and Initializing Variables
- Understanding Type Inference
- Using Strings and String Operations
- Exploring Collections in Dart: Lists
- Using the Spread and Null-aware Spread Operators
- Exploring Collections in Dart: Sets and Maps
- Using the Conditional If Block
- Using For and While Loops
- Using Enums and Switch Statements
- Defining Nullable and Non-nullable Types
- Using the Null Assertion Operator
- Using Null Aware Operators
- Implementing Late and Lazy Initialization
- Using Positional and Named Parameters
- Using Required Named Parameters and Default Values
- Passing First-class Functions
- Course Summary
Flutter Development: Using Classes & Objects in Dart
Course: 1 Hour, 54 Minutes
- Course Overview
- Using Initialization Lists and Parameters
- Using Named and Constant Constructors
- Using Factory Constructors and Private Variables
- Implementing Properties Using Getters and Setters
- Overloading Operators
- Implementing Callable Classes
- Introducing Inheritance
- Implementing Interfaces
- Extending Classes Using Extension Methods
- Implementing Mixin-based Inheritance
- Exploring Packages on pub.dev
- Installing and Using Packages
- Implementing Asynchronous Processing
- Using async and await Keywords
- Course Summary
Flutter Development: Getting Set Up with Flutter for Application Development
Course: 1 Hour, 10 Minutes
- Course Overview
- Installing Android Studio on MacOS
- Installing the Flutter SDK on MacOS
- Installing Android Studio on Windows
- Installing the Flutter SDK on Windows
- Running a Flutter Sample App Using the Console
- Running a Flutter Sample App Using Android Studio
- Running a Flutter App on an Android Emulator
- Running a Flutter Sample App Using the iOS Simulator
- Building a Simple Flutter Web Application
- Course Summary
Layouts in Flutter: Implementing Basic Flutter Layouts
Course: 1 Hour, 30 Minutes
- Course Overview
- Using Widgets in Flutter
- Rendering and Layout in Flutter
- Setting up a Flutter Project and Android Emulator
- Running our First Flutter App
- Using the Material App and Stateless Widgets
- Creating a Simple Layout Using Containers
- Exploring Container Properties
- Using Containers, Columns, and Images
- Assigning Themes to the Material App
- The Drawer and Bottom App Bar
- Course Summary
Layouts in Flutter: Exploring Stateful Widgets
Course: 1 Hour, 21 Minutes
- Course Overview
- Introducing Stateful Widgets
- Accessing the Same State with Multiple Widgets
- Changing State Using a Timer
- Building a Checkbox and Icon Widget with State
- Introducing the Row Widget
- Using Main Axis and Cross Axis for the Row Widget
- Exploring the Column Widget and its Properties
- Creating Expanded Widgets and Using the Flex Factor
- Exploring Issues with Inflexible Fixed-size Layouts
- Making Layouts Flexible Using the Flexible Widget
- Course Summary
Layouts in Flutter: Implementing Stacks, Lists, & Grids in Flutter
Course: 1 Hour, 35 Minutes
- Course Overview
- Introducing the Stack
- Creating Non-positioned and Positioned Widgets
- Introducing the ListView Widget
- Using List Tiles
- Building Infinite Lists with the ListView Builder
- Using a Dismissible Widget to Delete List Items
- Introducing the GridView Widget
- Displaying Images Using the GridView
- Configuring GridView Properties
- Building an Infinite Scrolling GridView
- Setting up a GridView to Hold Selection State
- Course Summary
Layouts in Flutter: Building an Application Using Layouts & Navigation
Course: 1 Hour, 38 Minutes
- Course Overview
- Configuring App Navigation with the Navigator Class
- Performing Navigation Using Named Routes
- Performing Navigation Using onGenerateRoute
- Exploring the Basic Structure of a Simple App
- Exploring the Drawer and Simple Screens in an App
- Exploring the Categories and Products Pages
- Understanding the Product, Item, and Cart Pages
- Building a Sample App Using Cupertino Widgets I
- Building a Sample App Using Cupertino Widgets II
- Configuring the Icon and App Name
- Running our App on a Real Device
- Course Summary
Assessment:
- Final Exam: Fundamentals of Dart Programming and Flutter Framework
Track 2: Building Mobile App with Flutter
Courses (10 hours +)
Forms and Animations with Flutter: Working with Input Widgets & Forms
Course: 1 Hour, 52 Minutes
- Course Overview
- Input Widgets and Forms in Flutter
- Displaying Text Fields
- Handling Updates to the Text Field
- Processing Input Using Radio Buttons
- Handling Input Using Checkboxes
- Toggling Values Using the Switch Input
- Selecting Input Values Using Dropdowns
- Working with Grouped Input Widgets
- Introducing Forms and Form Fields1
- Specifying Validators for Form Fields
- Configuring the Autovalidate Mode
- Creating a Custom Form Field1
- Using Custom State with a Custom Form Field
- Course Summary
Forms and Animations with Flutter: Detecting & Handling Gestures
Course: 1 Hour, 17 Minutes
- Course Overview
- Gestures in Flutter
- Handling Tap Gestures
- Handling Long Press and Double Tap Gestures
- Detecting Horizontal and Vertical Drags
- Accessing Drag Coordinates and Drag Velocity
- Using Dismissible Widgets to Delete List Elements
- Using Multiple Gesture Detectors
- Using Nested Gesture Detectors
- Disambiguating Gestures Using Raw Gesture Detectors
- Course Summary
Forms and Animations with Flutter: Implementing & Configuring Animations
Course: 2 Hours, 9 Minutes
- Course Overview
- Animations in Flutter
- Playing with Manual Animations
- Progressing Towards Implicit Animations
- Building Implicit Animations with AnimatedContainer
- Configuring Animation Curves
- Controlling Multiple Animations
- Using AnimatedOpacity and AnimatedCrossFade
- Playing with the AnimatedSlide5
- Using the Animation Controller
- Using the AnimatedBuilder
- Creating Animations using Tweens
- Using Tween Animations in a Real-world Scenario
- Implementing Staggered Animations
- Using Hero Animations for Smooth Transitions
- Using Hero Animations for Grid Elements
- Implementing Physics Simulations
- Using Spring Simulations for a Draggable Card
- Course Summary
Flutter and Backends: Connecting to Remote Backends Using HTTP Requests
Course: 1 Hour, 33 Minutes
- Course Overview
- Data Persistence in Flutter
- Viewing the Fake Store API REST Endpoints
- Making an HTTP GET Request
- Decoding and Parsing JSON Strings
- Improving the App Using Custom Fonts and Ratings
- Parsing JSON in the Background Using Isolates
- Adding Search Functionality to the App
- Setting up an HTTP Server Using Dart
- Making HTTP GET Requests to the Local Server
- Making HTTP POST Requests to the Local Server
- Making HTTP DELETE Requests to the Local Server
- Making HTTP PUT Requests to the Local Server
- Course Summary
Flutter and Backends: Persisting Data on the Local Device
Course: 2 Hours, 11 Minutes
- Course Overview
- Local Persistence in Flutter
- Reading Text Data Using the Flutter Services Library
- Reading and Parsing Contents of a CSV File
- Accessing Files in the App Documents Directory
- Listing Files Present on the Local Device
- Creating, Editing, and Deleting Files
- Setting up Access to SharedPreferences
- Retrieving Key Value Pairs Using SharedPreferences
- Storing the Last Search Term in SharedPreferences
- Using SharedPreferences for Autocomplete
- Storing User Preferences in an Application
- Setting up a Database Helper to Work With SQLite
- Viewing Products Retrieved from the SQLite Database
- Adding Products to the SQLite Database
- Deleting and Updating Products in the SQLite Database
- Course Summary
Flutter and Backends: Integrating Flutter with Google Firebase
Course: 1 Hour, 53 Minutes
- Course Overview
- Application State Management in Flutter
- Using Providers for State Management
- Implementing the Shopping Cart Using Providers
- Introduction to Firebase
- Integrating with Firebase
- Setting up Document Collections in Cloud Firestore
- Using Stream Builders for Realtime Updates
- Adding a Splash Screen
- Setting up the Login Registration and Profile Page
- Setting up the Bottom Navigation Bar
- Implementing a Drawer and Edit Profile Page
- Wiring up the Favorites Page
- Wiring up the Cart and Orders Pages
- Configuring Security Rules for Cloud Firestore
- Course Summary
Mobile Development: Testing & Debugging Flutter Applications
Course: 1 Hour, 53 Minutes
- Course Overview
- Flutter App Testing
- Using the Android Studio IDE to Run Unit Tests
- Running Unit Tests Using the Command Line
- Generating Mocks Using Mockito
- Setting Up Complex Unit Tests Using Mocks
- Using Widget Tester Finders and Matchers
- Setting Up Unit Tests for Stateful Widgets
- Testing Scrollable Containers
- Performing a Unit Test on Animated Widgets
- Testing Dismissible Widgets
- Flutter App Debugging
- Viewing Flutter Inspector Widgets
- Debugging Flutter Apps Using DevTools
- Course Summary
Assessment:
- Final Exam: Building Mobile Apps with Flutter
Language | English |
---|---|
Qualifications of the Instructor | Certified |
Course Format and Length | Teaching videos with subtitles, interactive elements and assignments and tests |
Lesson duration | 23 Hours |
Assesments | The assessment tests your knowledge and application skills of the topics in the learning pathway. It is available 365 days after activation. |
Online Virtuele labs | Receive 12 months of access to virtual labs corresponding to traditional course configuration. Active for 365 days after activation, availability varies by Training |
Online mentor | You will have 24/7 access to an online mentor for all your specific technical questions on the study topic. The online mentor is available 365 days after activation, depending on the chosen Learning Kit. |
Progress monitoring | Yes |
Access to Material | 365 days |
Technical Requirements | Computer or mobile device, Stable internet connections Web browsersuch as Chrome, Firefox, Safari or Edge. |
Support or Assistance | Helpdesk and online knowledge base 24/7 |
Certification | Certificate of participation in PDF format |
Price and costs | Course price at no extra cost |
Cancellation policy and money-back guarantee | We assess this on a case-by-case basis |
Award Winning E-learning | Yes |
Tip! | Provide a quiet learning environment, time and motivation, audio equipment such as headphones or speakers for audio, account information such as login details to access the e-learning platform. |
There are no reviews written yet about this product.
OEM Office Elearning Menu Genomineerd voor 'Beste Opleider van Nederland'
OEM Office Elearning Menu is trots genomineerd te zijn voor de titel 'Beste Opleider van Nederland' door Springest, een onderdeel van Archipel. Deze erkenning bevestigt onze kwaliteit en toewijding. Hartelijk dank aan al onze cursisten.
Reviews
There are no reviews written yet about this product.