Programming in C++ (version 20) Training
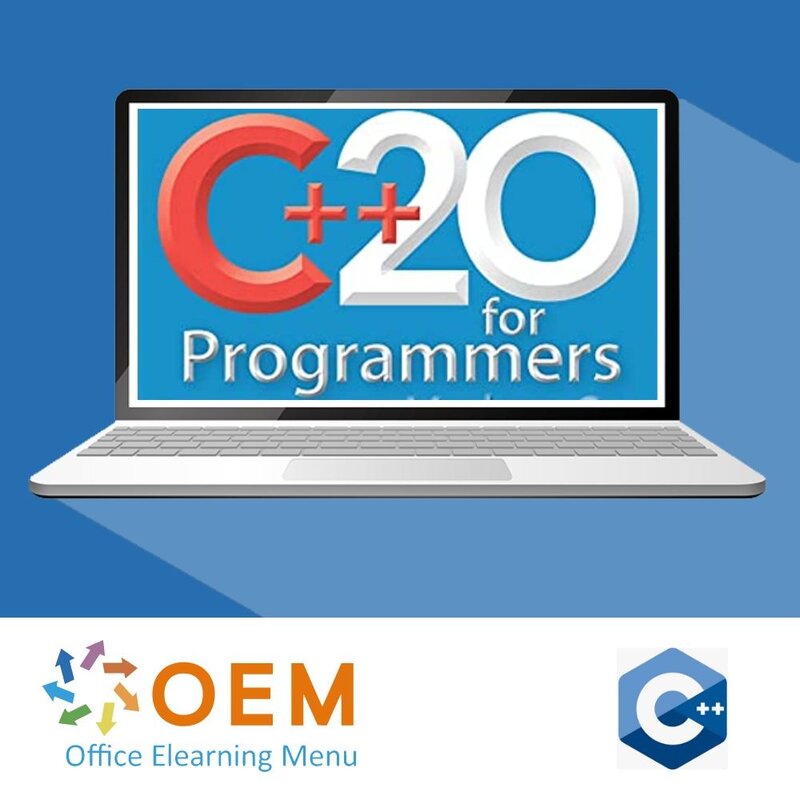
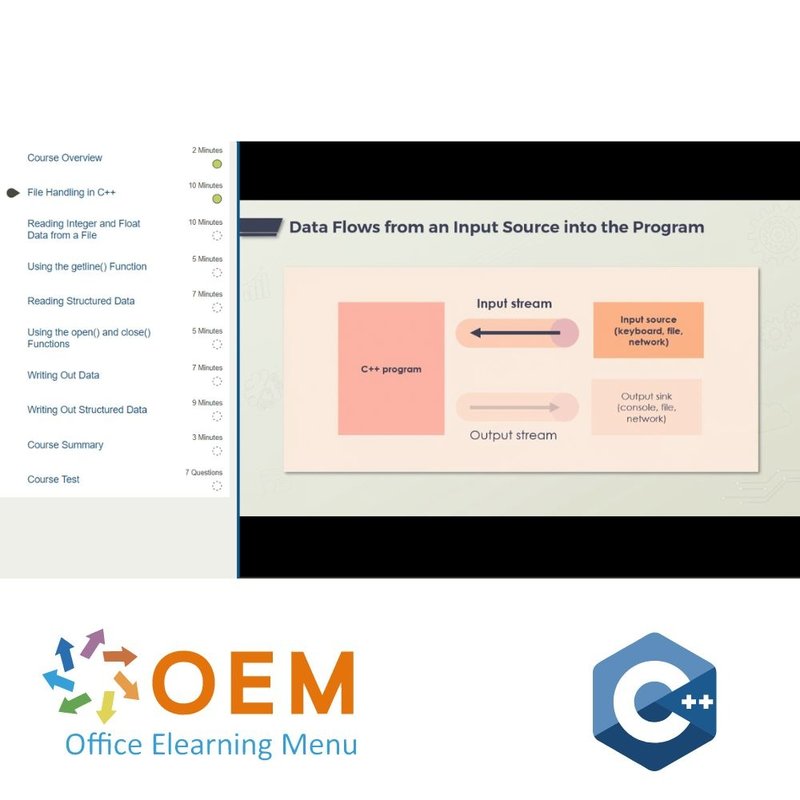
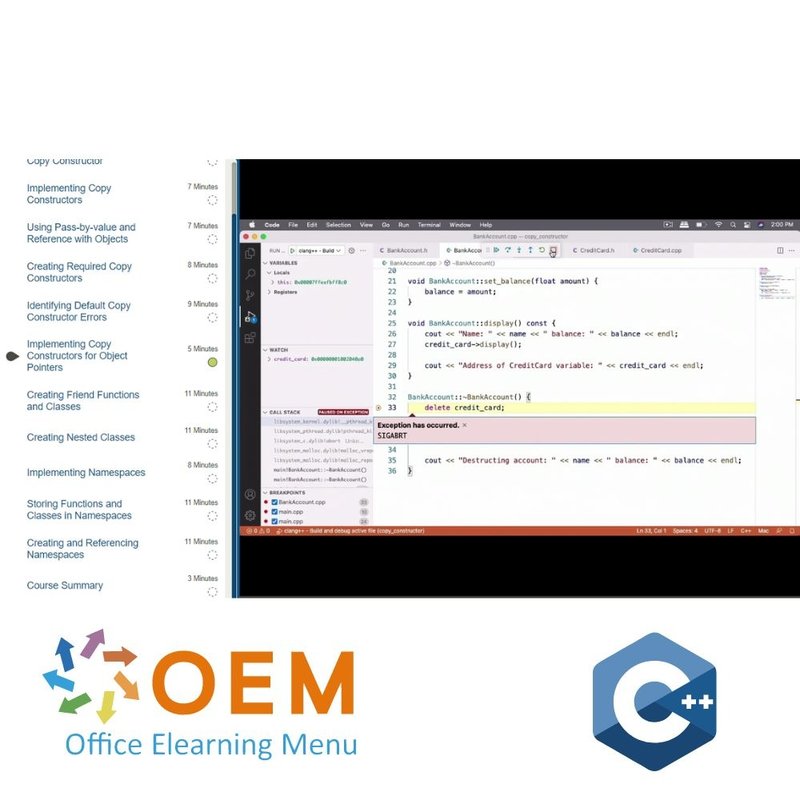
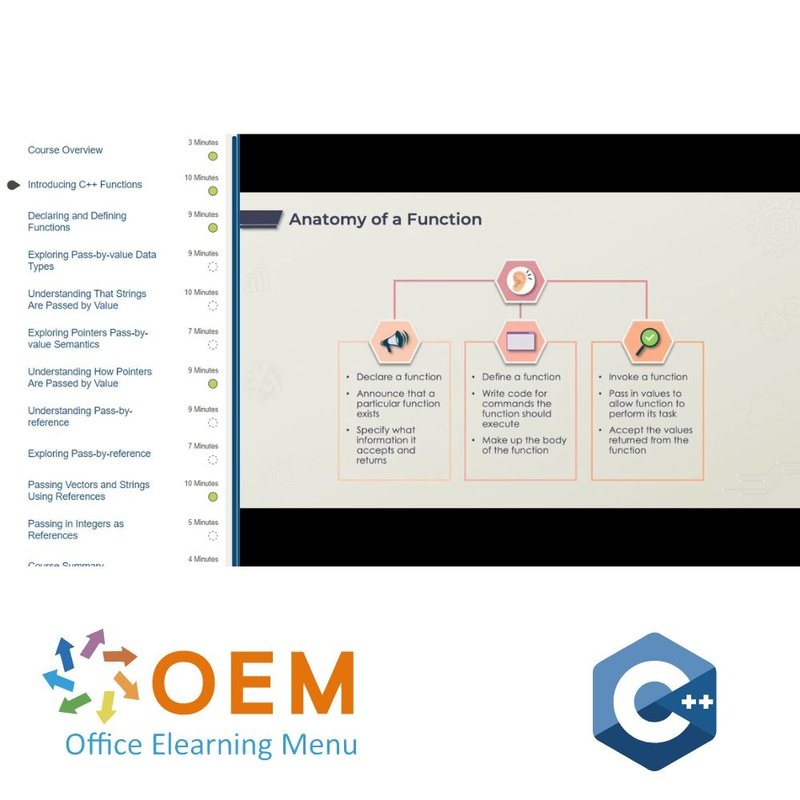
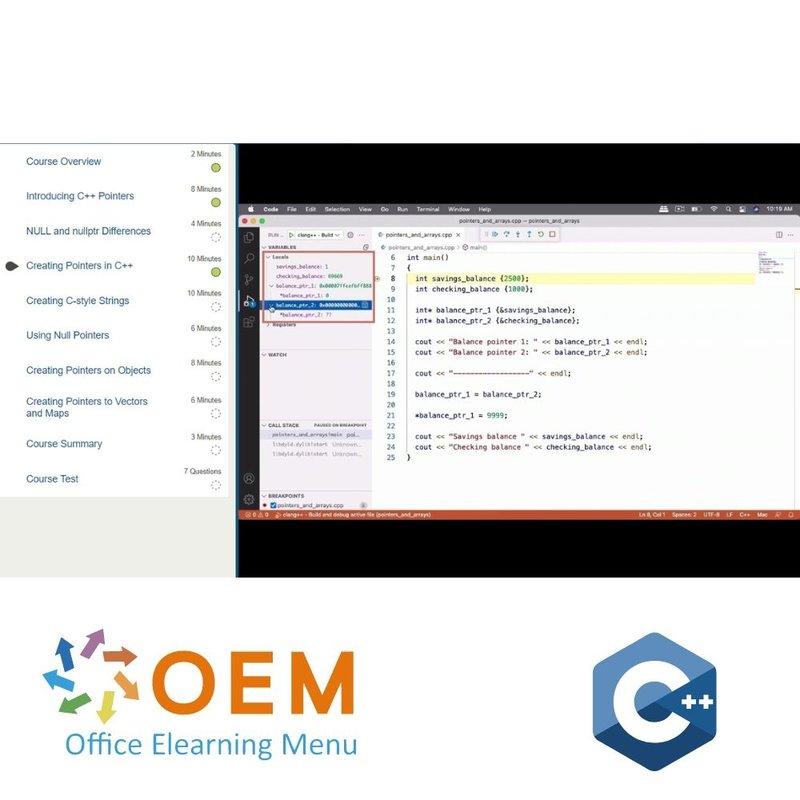
Programming in C++ (version 20) Training
Programming in C++ (version 20) Training Award-winning E-Learning course Extensive interactive videos with spoken text Certified teachers Practical exercises Certificate.
Read more- Discounts:
-
- Buy 2 for €194,04 each and save 2%
- Buy 3 for €192,06 each and save 3%
- Buy 5 for €184,14 each and save 7%
- Buy 10 for €178,20 each and save 10%
- Buy 25 for €168,30 each and save 15%
- Buy 50 for €154,44 each and save 22%
- Buy 100 for €138,60 each and save 30%
- Buy 200 for €99,00 each and save 50%
- Availability:
- In stock
- Delivery time:
- Ordered before 5 p.m.! Start today.
- Award Winning E-learning
- Lowest price guarantee
- Personalized service by our expert team
- Pay safely online or by invoice
- Order and start within 24 hours
Programming in C++ (version 20) E-Learning
C++ is still a powerful programming language, despite the advent of various modern languages. Learning C++ enables the learner to easily perceive the most trending programming languages The Programming C++ LearningKit facilitates the learners to understand the functional programming model and Object-oriented programming model and to identify which model to apply under what circumstances. Each learning path presents adequate chances for writing programs and identifying & fixing errors. This LearningKit is a blend of all the fundamentals and the new features of C++. The intent here is to provide the learners with all the most crucial information in one place to write correct, portable, professional-quality code.
This LearningKit with more than 56 hours of learning is divided into three tracks:
Course content
TRACK 1: GETTING STARTED WITH C++
In this track, the focus will be on the fundamental features of the C++ programming language.
Courses (20 hours +):
Fundamentals of C++: Getting Started
Course: 1 Hour, 30 Minutes
- Course Overview
- C++ Introduction
- Principles of C++ Code Execution
- C++ Code Principles
- C++'s Compilation Process
- C and C++ Differences
- Exploring Features of C++ and Its Compilers
- Setting up the G++ Compiler
- Executing C++ on Windows
- Setting up C++ on Mac
- Running C++ Code with a Build
- Printing Text Using C++
- Course Summary
Fundamentals of C++: Using Variables & Datatypes
Course: 1 Hour, 38 Minutes
- Course Overview
- Introducing C++ Variables
- Exploring Numeric Data Types
- Using Integral, Character, and Floating-point Types
- Creating Boolean Variables in C++
- Performing Logical and Arithmetic Operations
- Creating C-style Strings
- Creating C++-style Strings
- Modifying Strings
- Using Member Functions with Strings
- Indexing into Strings
- Using Operators with Strings
- Course Summary
Fundamentals of C++: Using the auto Keyword, Enums, and I/O Streams
Course: 55 Minutes
- Course Overview
- Using the auto Keyword
- Exploring Enumerated Types
- Accepting Single-word Input
- Experimenting with Accepting Input
- Exploring Finer Points of Accepting Input
- Formatting Data Using Utility Functions
- Course Summary
Control Structures in C++: Using Conditional Control Structures
Course: 1 Hour, 38 Minutes
- Course Overview
- Understanding Control Structures
- Creating if-blocks and if-else-if Ladders
- Using Overloaded Relational Operators
- Introducing Vectors
- Creating Iterators on Vectors
- Creating Variables in an if-block Scope
- Using Logical Operators
- Creating Switch Statements
- Creating Variables in the Scope of Switch Statements
- Creating Cases Based on Strings in Switch Statements
- Exploring the Finer Points of Switch Statements
- Creating Switch Statements on Strings
- Course Summary
Control Structures in C++: Range-based for Loops
Course: 1 Hour, 37 Minutes
- Course Overview
- Introducing Arrays and For Loops
- Using the Break and Continue Keywords
- Iterating over Multidimensional Arrays
- Understanding the STL
- Accessing Out-of-bound Elements of a Vector
- Searching in a Vector Using Templates
- Using the cend(), rend(), and crend() Functions
- Debugging C++ Code
- Configuring the Debugging of Code
- Using Debuggers to Play the Execution of Code
- Using String References
- Creating Range-based For Loops
- Course Summary
Pointers and References in C++: Getting Started with Pointers
Course: 58 Minutes
- Course Overview
- Introducing C++ Pointers
- NULL and nullptr Differences
- Creating Pointers in C++
- Creating C-style Strings
- Using Null Pointers
- Creating Pointers on Objects
- Creating Pointers to Vectors and Maps
- Course Summary
Pointers and References in C++: Allocating Memory with New & Delete Operators
Course: 1 Hour, 25 Minutes
- Course Overview
- Understanding the Use of Pointers
- Allocating Memory in C++
- Allocating Memory Using the new Keyword
- Understanding Finer Points of New and Delete
- Creating Strings and Vectors on the Heap
- Allocating Memory for Arrays on the Heap
- Exploring Finer Points of Array New and Delete
- Const Pointers versus Pointers to Consts
- Using Constant Variables and Pointers
- Creating Pointers to Constants
- Creating Constant Pointers
- Course Summary
Pointers and References in C++: Using Smart Pointers in Modern C++
Course: 1 Hour, 39 Minutes
- Course Overview
- Introduction to Smart Pointers
- Types of Smart Pointers
- Introducing Unique Pointers
- Exploring Unique Pointers
- Creating Shared Pointers
- Exploring Shared Pointers
- Exploring Finer Points of Shared Pointers
- Creating Weak Pointers
- Exploring Finer Points of Weak Pointers
- Exploring Invalid Weak Pointers
- Understanding the Use Case of Weak Pointers
- Course Summary
Pointers and References in C++: Working with References
Course: 46 Minutes
- Course Overview
- Introduction to References
- Creating References
- Referencing Different Objects
- Modifying Data Using References
- Creating Constant References
- Casting Variables to Be Constant
- Course Summary
Functions in C++: Using Functions & Parameter Passing
Course: 1 Hour, 32 Minutes
- Course Overview
- Introducing C++ Functions
- Declaring and Defining Functions
- Exploring Pass-by-value Data Types
- Understanding That Strings Are Passed by Value
- Exploring Pointers Pass-by-value Semantics
- Understanding How Pointers Are Passed by Value
- Understanding Pass-by-reference
- Exploring Pass-by-reference
- Passing Vectors and Strings Using References
- Passing in Integers as References
- Course Summary
Functions in C++: Using Default Arguments & Function Overloading
Course: 57 Minutes
- Course Overview
- Using Default Arguments
- Returning Pointers and References
- Using the auto Keyword
- Overloading Functions
- Exploring the Nuances of Overloading Functions
- Creating Header Files
- Course Summary
Assessment:
Final Exam: Getting Started in C++
TRACK 2: OBJECT-ORIENTED CONCEPTS IN C++
In this track, the focus will be on core programming in C++, where the learners will recognize the significance of object-oriented programming.
Courses (23 hours +)
OOP in C++: Getting Started with Object-oriented Programming
Course: 1 Hour, 25 Minutes
- Course Overview
- Object-oriented Programming (OOP)
- The Structure of Object-oriented Programming (OOP)
- Member Variables and Functions
- Creating Objects of a Class
- Defining Getter and Setter Functions
- Creating Constructors and Instantiating Objects
- Utilizing the Private Access Modifier
- Creating a Custom Header File
- Working with Custom Header Files in Code
- Discerning Common Errors When Using Header Files
- Course Summary
OOP in C++: Instantiating Objects Using Constructors
Course: 50 Minutes
- Course Overview
- Adding Default Arguments to Constructors
- Initializing Variables Using Initialization Lists
- Distinguishing the Nuances of Initialization Lists
- Execute Constructor Chaining
- Implementing the explicit Keyword
- Creating Structs in C++
- Course Summary
OOP in C++: Using the this Pointer & const Members
Course: 1 Hour, 5 Minutes
- Course Overview
- Using the this Pointer
- Chaining Method Invocations Using the this Pointer
- Creating const Objects and const Member Functions
- Identifying Common const Method Errors
- Using const Pointers and References
- Overloading Functions as const and Non-const
- Returning const and Non-const Values from Functions
- Using the const_cast and mutable Keywords
- Course Summary
OOP in C++: Using Static Members & Destructors
Course: 56 Minutes
- Course Overview
- Creating Pre-C++17 Static Variables
- Creating C++17 Static Variables
- Accessing Static Variables through Classes
- Defining and Invoking Static Functions
- Defining and Using Destructors
- Implementing Constructors and Destructors
- Instantiating and Deallocating a Pointer
- Course Summary
OOP in C++: Copy Constructors, Nested Classes, & Namespaces
Course: 1 Hour, 43 Minutes
- Course Overview
- Creating Copies Using a Copy Constructor
- Implementing Copy Constructors
- Using Pass-by-value and Reference with Objects
- Creating Required Copy Constructors
- Identifying Default Copy Constructor Errors
- Implementing Copy Constructors for Object Pointers
- Creating Friend Functions and Classes
- Creating Nested Classes
- Implementing Namespaces
- Storing Functions and Classes in Namespaces
- Creating and Referencing Namespaces
- Course Summary
C++ Inheritance & Polymorphism: Using Inheritance for is-a Relationships
Course: 1 Hour, 31 Minutes
- Course Overview
- Inheritance
- Inheritance and Composition
- Using Public Inheritance
- Using Members of Classes in Inheritance Hierarchies
- Accessing Members of Base Classes from Child Classes
- Using the 'protected' Access Modifier
- Access Modifiers
- Access Modifiers with Inheritance
- Using the Base Class Access Specifier
- Using 'protected' as the Base Class Access Specifier
- Using Private Inheritance
- Bypassing Access Modifiers Using 'using'
- Course Summary
C++ Inheritance & Polymorphism: Constructors, Destructors, & Inheritance
Course: 1 Hour, 24 Minutes
- Course Overview
- Using Constructors in Inheritance Hierarchies
- Using Base Class Constructors in Child Classes
- Accessing Base Class Fields from the Child Class
- Using Base Class Constructors in Derived Classes
- Using Copy Constructors in Inheritance
- Using Destructors in Inheritance
- Setting up an Inheritance Hierarchy for Polymorphism
- Creating Pointers with Inheritance
- Creating References with Inheritance
- Course Summary
C++ Inheritance & Polymorphism: Understanding & Using Polymorphism
Course: 2 Hours, 4 Minutes
- Course Overview
- Understanding Name Hiding
- Exploring Finer Points of Name Hiding
- Understanding How Static Binding Can Be Dangerous
- Polymorphism
- Mechanics of Polymorphism
- Introducing the 'virtual' Keyword
- Exploring Runtime Polymorphism
- Using Runtime Polymorphism with References
- Viewing Resources Used in Dynamic Method Dispatch
- Exploring Problems Related to Runtime Polymorphism
- Understanding Covariance
- Understanding the 'override' Specification
- Using the 'final' Keyword
- Course Summary
C++ Inheritance & Polymorphism: Pure Virtual Functions & Abstract Classes
Course: 1 Hour, 22 Minutes
- Course Overview
- Using Virtual Destructors
- Understanding Object Slicing
- Exploring Default Arguments to Virtual Functions
- Using Virtual Methods in Constructors & Destructors
- Exploring the '::' Operator
- Using Pure Virtual Functions
- Exploring Abstract Classes
- Using Interfaces
- Course Summary
C++ Inheritance & Polymorphism: Multiple Inheritance & the Diamond Hierarchy
Course: 1 Hour, 22 Minutes
- Course Overview
- Multiple Inheritance and Diamond Hierarchy Overview
- Understanding Multiple Inheritance
- Implementing Virtual Methods in Multiple Inheritance
- Creating a Diamond Inheritance Hierarchy
- Using C-style Casts in C++
- Performing Static Casts
- Casting Objects
- Performing Dynamic Casts
- Exploring Errors Related to Dynamic Casts
- Course Summary
C++: Getting Started with Operator Overloading
Course: 1 Hour, 23 Minutes
- Course Overview
- Operator Overloading in C++
- Creating Classes in C++s
- Overloading Operators
- Using Code to Overload Operators
- Using Overloaded Operators as Member Functions
- Using Non-member Functions to Overload Operators
- Using Friend Functions with Operator Overloading
- Overloading Operators with Primitive Right Operands
- Using Best Practices of Operator Overloading
- Overloading Operators with Primitive Left Operands
- Course Summary
C++: Overloading Arithmetic & Relational Operators
Course: 1 Hour, 46 Minutes
- Course Overview
- Using std::rel_ops
- Invoking Operators from Other Operators
- Generating Operator Overloads using std::rel_ops
- Overloading the + Operator
- Using the Overloaded + Operator
- Identifying Requirements for + Operator Overloads
- Overloading the += Operator
- Using the Overloaded += Operator
- Identifying Requirements for += Operator Overloads
- Overloading the Pre and Post Increment Operators
- Using the Overloaded ++ Operator
- Course Summary
C++: Stream Operators, Assignment Operators, & Copy-and-Swap
Course: 1 Hour, 25 Minutes
- Course Overview
- Using Insertion and Extraction Operators
- Using the << and >> Operators as Functions
- Overloading Insertion and Extraction Operators
- Using the Copy Assignment Operator
- Implementing the Copy Assignment Operator
- Comparing Copy Constructor to Copy Assignment
- Exploring Default Assignment Operator Issues
- Overloading the Copy Assignment Operator
- Using the Copy-Swap Idiom
- Creating Copy Assignment Operations with Copy-Swap
- Course Summary
C++: Using Function Templates
Course: 1 Hour, 47 Minutes
- Course Overview
- Function and Class Templates
- Defining the Use Case of Function Templates
- Recognizing the Finer Points of Function Templates
- Specifying Types for Templates
- Defining Templated Functions in Header Files
- Using Templated Functions in .cpp Files
- Using Function Templates with Custom Types
- Demonstrating Issues of Pointers with Templates
- Defining Template Specializations
- Using Template Specializations
- Overloading Templated Functions
- Overloading Templated Functions with Pointer Types
- Course Summary
C++: Function Templates with Multiple Parameters & Non-type Parameters
Course: 40 Minutes
- Course Overview
- Using Multi-value Function Templates
- Specifying Function Return Types through Templates
- Using decl_type
- Using Default Values in Template Parameters
- Using Non-type Template Parameters
- Course Summary
C++: Using Class Templates
Course: 1 Hour, 52 Minutes
- Course Overview
- Using Class Template Parameters
- Creating Classes with Template Parameters
- Using the Braced Initialization List Constructor
- Automatically Deducing Class Template Parameters
- Using Functional Notation for Initializing Variables
- Using Non-type Template Parameters with Classes
- Demonstrating Features of Non-type Parameters
- Specializing Class Templates
- Using Specialized Templates
- Using Partial Specializations
- Performing Partial Specializations
- Using static_assert
- Defining Friend Functions to Templated Classes
- Course Summary
C++: Using the Move Constructor & Move Assignment Operator
Course: 1 Hour, 23 Minutes
- Course Overview
- Contrasting L-Values and R-Values
- Using L-Value and R-Value References
- Implementing the Rule of
- Illustrating the Need for R-value References
- Illustrating the Advantages of Move Constructors
- Using Move Assignment Operators
- Outlining Features of the Move Assignment Operator
- The RAII Paradigm
- Using RAII and the Rule of
- Course Summary
TRACK 3: ADVANCED CONCEPTS IN C++
In this track, the focus will be on the exploration of advanced C++ topics like File Handling, Data Structures, First-class functions, Templates, C++ Lambdas, Graphics, and the development of a simple gaming application.
Courses (12 hours +)
File Handling in C++: Working with File Streams
Course: 58 Minutes
- Course Overview
- File Handling in C++
- Reading Integer and Float Data from a File
- Using the getline() Function
- Reading Structured Data
- Using the open() and close() Functions
- Writing Out Data
- Writing Out Structured Data
- Course Summary
File Handling in C++: Performing Advanced File Stream Operations
Course: 1 Hour, 55 Minutes
- Course Overview
- Writing Out Custom Objects
- Reading in Custom Objects
- Reviewing Operations with Input Streams
- Using get() and getline()
- Using seekg()
- Using put(), read(), and write()
- Understanding the Write and Append Modes
- Using the Ate Mode
- Writing Out Data Using Binary Mode
- Write Out and Read in Binary Data
- Reviewing Stream State Bits
- Using the Filesystem Library
- Performing Operations on Files
- Course Summary
Exception Handling in C++
Course: 1 Hour, 48 Minutes
- Course Overview
- Techniques for Propagating Errors
- How Exceptions Are Thrown and Handled
- Throwing and Handling Exceptions
- Defining Multiple Catch Blocks
- Handling Exceptions Using the try-catch Block
- Throwing Exceptions Using Exception Classes
- Creating and Using Custom Exception Classes
- Using Custom Exceptions and Catch Blocks
- Catching Exceptions by Reference
- Violating the noexcept Keyword
- Cascading Errors up the Stack Trace
- Using the Function-try-block
- Course Summary
C++: Working with Sequence Containers
Course: 1 Hour, 26 Minutes
- Course Overview
- Associative and Sequence Containers
- Sequence Container Types
- Creating and Iterating Arrays
- Using Vectors
- Inserting, Modifying, and Removing Vector Elements
- Creating Forward Lists
- Generating Lists
- Queue, Stack, and Priority Queue Container Adapters
- Making Deques
- Creating and Using Container Adapters
- Course Summary
C++: Working with Associative Containers & Algorithms
Course: 1 Hour, 22 Minutes
- Course Overview
- Associative Containers
- Making and Using Regular Sets
- Using Unordered Sets and Multisets
- Creating and Iterating Maps
- Utilizing Unordered Maps
- Creating Multimaps
- Ordering Containers
- Using Algorithms on Containers
- Performing Advanced Algorithms and Transformations
- Course Summary
First-class Functions in C++: Defining & Using First-class Functions
Course: 1 Hour, 9 Minutes
- Course Overview
- First-class Functions in C++
- Creating Function Pointers
- Invoking Functions through Function Pointers
- Returning Functions from Other Functions
- Using Typedefs and Type Aliases
- Passing Functions in as Input Arguments
- Utilizing Templates with Higher-order Functions
- Creating and Using Functors
- Using Functors with Templates
- Course Summary
First-class Functions in C++: Using Lambdas & Closures
Course: 55 Minutes
- Course Overview
- Lambda Expressions
- Creating Lambdas
- Passing Lambdas into Functions
- Using Lambdas with STL Algorithms
- Lambda Closures
- Capturing Variables within Lambdas
- Capturing Variables by Reference
- Course Summary
C++: Introduction to Graphics with SFML
Course: 1 Hour, 35 Minutes
- Course Overview
- Simple and Fast Multimedia Library (SMFL)
- Installing SFML on macOS
- Installing SFML on Windows
- Opening and Managing Windows
- Setting Window Icons and Window Properties
- Handling Focus Events and Keyboard Events
- Handling Text Entered and Mouse Events
- Drawing Text
- Drawing Rectangles and Circles
- Drawing Polygons
- Course Summary
C++: Creating a Complete Game in SFML
Course: 1 Hour, 33 Minutes
- Course Overview
- Drawing Textures and Sprites
- Drawing Using Vertex Arrays
- Moving, Rotating, and Scaling Entities
- Using Sprite Sheets for Animation
- Interacting with the World Using Views
- Playing Music in Games
- Tic-Tac-Toe: Defining the Game
- Tic-Tac-Toe: Implementing the Game
- Tic-Tac-Toe: Playing the Game
- Course Summary
Language | English |
---|---|
Qualifications of the Instructor | Certified |
Course Format and Length | Teaching videos with subtitles, interactive elements and assignments and tests |
Lesson duration | 56 Hours |
Assesments | The assessment tests your knowledge and application skills of the topics in the learning pathway. It is available 365 days after activation. |
Online Virtuele labs | Receive 12 months of access to virtual labs corresponding to traditional course configuration. Active for 365 days after activation, availability varies by Training |
Online mentor | You will have 24/7 access to an online mentor for all your specific technical questions on the study topic. The online mentor is available 365 days after activation, depending on the chosen Learning Kit. |
Progress monitoring | Yes |
Access to Material | 365 days |
Technical Requirements | Computer or mobile device, Stable internet connections Web browsersuch as Chrome, Firefox, Safari or Edge. |
Support or Assistance | Helpdesk and online knowledge base 24/7 |
Certification | Certificate of participation in PDF format |
Price and costs | Course price at no extra cost |
Cancellation policy and money-back guarantee | We assess this on a case-by-case basis |
Award Winning E-learning | Yes |
Tip! | Provide a quiet learning environment, time and motivation, audio equipment such as headphones or speakers for audio, account information such as login details to access the e-learning platform. |
There are no reviews written yet about this product.
OEM Office Elearning Menu Top 2 in ICT-trainingen 2024!
OEM Office Elearning Menu is trots op de tweede plaats in de categorie ICT-trainingen 2024 bij Beste Opleider van Nederland (Springest/Archipel). Dank aan al onze cursisten voor hun vertrouwen!
Reviews
There are no reviews written yet about this product.